JAVA was developed by Sun Microsystems Inc in 1991, later
acquired by Oracle Corporation. It was developed by James Gosling and Patrick
Naughton. It is a simple programming language.
Writing, compiling and debugging a program is easy in java. It helps to create modular programs and
reusable code.
Decimal to Binary conversion in java program
import java.util.Scanner;
public class dectobin
{
public static void main(String[] args)
{
int dec;
int rem;
String output="";
Scanner scan = new Scanner(System.in);
System.out.println("Enter the number");
dec = scan.nextInt();
while(dec>0)
{
rem = dec%2;
output = rem + output;
dec=dec/2;
}
System.out.println("the binary number is :
"+output);
}
}
The output screen shows:

2. Logic Function AND
The AND gate is a basic digital logic gate that
implements logical conjunction - it behaves according to the truth table to the
right. A HIGH output (1) results only if both the inputs to the AND gate are
HIGH (1). If neither or only one input to the AND gate is HIGH, a LOW output
results.
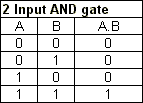
Sample java program for logic function AND
import java.util.Scanner;
public class logicAND {
public static void main(String args[]){
int a;
int b;
Scanner input = new Scanner(System.in);
System.out.println("Input A");
a = input.nextInt();
System.out.println("Input B");
b = input.nextInt();
if (a==1&&b==1){
System.out.println("The output is 1."); }
else if (a==0&&b==1) {
System.out.println("The output is 0."); }
else if (a==1&&b==0) {
System.out.println("The output is 0.");}
else if (a==0&&b==0) {
System.out.println("The output is 0.");}
else
{System.out.println("Invalid!"); }
}
}
Since the logic AND have a 4 possible output, this
condition will show if the users have a different input.
1st condition: if the user input for A is 1 and b is 1,
the output will be 1.
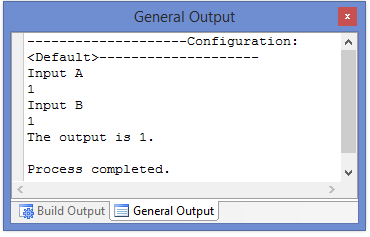
2nd condition: if the user input for A is 0 and B is 1,
the output will be 0;
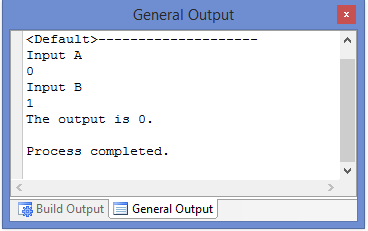
3rd condition: if the user input for A is 1 and B is 0,
the output will be 0;
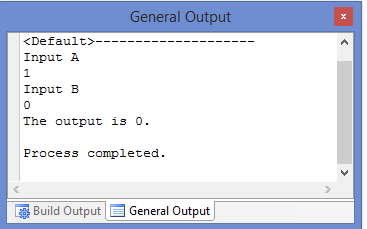
4th condition: if the user input for A is 0 and B is 0,
the output will be 0.
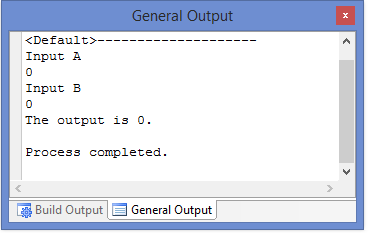
If the user’s input is not equal to 1 or 0, the output
will be “Invalid”, since in logic AND, it only have 1 and 0 input/output.
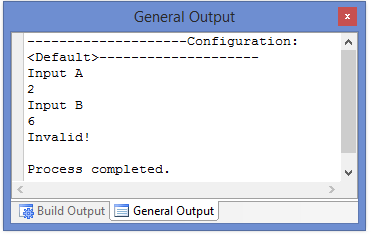
3. Binary to Decimal conversion
import java.util.Scanner;
public class binarytodec
{
public static void
main(String args[])
{
int binnum,
decnum=0, i=1, rem;
Scanner scan
= new Scanner(System.in);
System.out.print("Enter Binary Number : ")
binnum =
scan.nextInt();
while(binnum != 0)
{
rem =
binnum%10;
decnum
= decnum + rem*i;
i =
i*2;
binnum
= binnum/10;
}
System.out.print("Equivalent Decimal Value is :\n");
System.out.print(decnum);
}
}
sample output
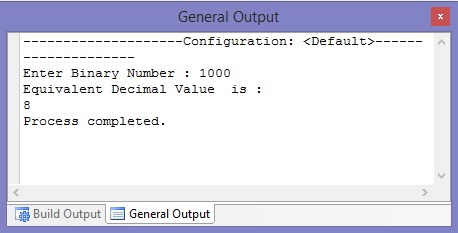
4. Power set in java program using concatenate.
import java.util.Scanner;
public class powerset {
public static void main(String[] args) {
String a,b,c;
Scanner input = new Scanner(System.in);
System.out.println("Enter your top three favorite
fruits:");
a = input.next();
b = input.next();
c = input.next();
System.out.println(" ");
System.out.print("This shows the set of
fruits(A):");
System.out.println("
"+"A"+"="+"{"+"("+a+","+b+","+c+")"+"}");
System.out.println(" ");
System.out.println("|P(S)|=2^n");
System.out.println("|P(S)|=2^3=8");
System.out.println(" ");
System.out.println("This shows the possible set of
Set A:");
System.out.println(" ");
System.out.println("
"+"A"+"="+"{"+"(empty
set)"+","+"("+a+")"+","+"("+b+")"+","+"("+c+")"
+","+"("+a+","+b+")"+
","+ "("+a+","+c+")" +
"("+b+","+c+")" +
"("+a+","+b+","+c+")");
}
}
sample output

5.Simple transaction java program
import java.util.Scanner;
public class transaction{
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String product;
float item;
float cost;
double pay;
double
discount;
double total;
int a=1;
int
purchase=1;
int yes;
System.out.println("Welcome Shoppers! ");
System.out.println(" ");
for
(a=1;a<=5;a++)
{
System.out.println("buy "+ purchase++ +" item and get a
"+a+ "% discount" );
}
do {
System.out.println("buy more items to avail our 10% discount "
);
System.out.println(" ");
System.out.print("ITEM: ");
product=input.next();
System.out.println(" ");
System.out.print("Quantity: ");
item=input.nextFloat();
System.out.println(" ");
System.out.print("Price : ");
cost=input.nextFloat();
System.out.println("
");
pay=item*cost;
System.out.println("Total :"+product+" = "+pay);
if (item==1)
{
discount=pay*0.01;
System.out.println("Less 1% Discount = "+discount);
total=pay-discount;
System.out.println("Total amount = " + product+" "+total);
}
else if
(item==2)
{
discount=pay*0.02;
System.out.println("Less 2% Discount = "+discount);
total=pay-discount;
System.out.println("Total amount = "+product+" "+total);
}
else if
(item==3)
{
discount=pay*0.03;
System.out.println("Less 3% Discount = "+discount);
total=pay-discount;
System.out.println("Total amount = "+product+" "+total);
}
else if (item==4)
{
discount=pay*0.04;
System.out.println("Less 4% Discount = "+discount);
total=pay-discount;
System.out.println("Total amount = " +product+" "+total);
}
else if
(item<=7)
{
discount=pay*0.05;
System.out.println("Less 5% Discount = "+discount);
total=pay-discount;
System.out.println("Total
amount = "+product+" "+total);
}
else if
(item>=8)
{
discount=pay*0.10;
System.out.println(" You avail our 10% discount = " + discount);
total=pay-discount;
System.out.println("Total
amount :" + product+" = "
+total);
}
System.out.println(" ");
System.out.println(" Would you like to purchase another one?
");
System.out.println(" ");
System.out.println("Please press 1 if YES ");
System.out.println("Please press 2 if NO ");
yes=input.nextInt();
}
while(yes==1);
System.out.println("Thank you for
shopping! ");
System.out.println("Please come again.");
}
}
sample output
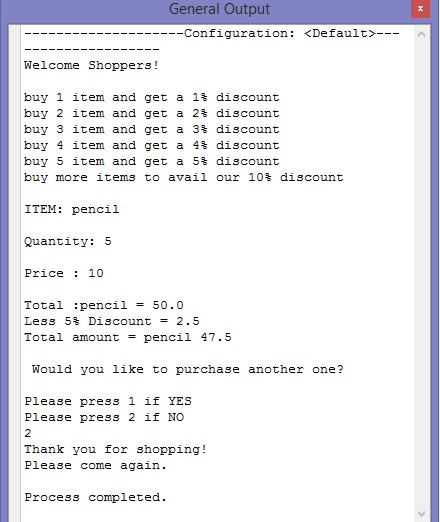
Finding the square, square root, cube root of a integer in java.
import java.util.*;
/*Java Program to find square, square root and cube of a given number*/
public class sqrt
{
public static void main(String args[])
{
Scanner sc=new
Scanner(System.in);
int num=0;
int a=0;
double I=0;
double c=0;
System.out.println("Enter an integer:");
num=sc.nextInt();
a=num+1;
System.out.println("Integer"+" "+"Square"+" "+"Sqrt"+" "+"Cube");
System.out.println("----------------------------------------------------");
for (num=1;a<=25;a++)
{
System.out.print(" "+a+" ");
System.out.print(Math.round( Math.pow(a, 2))+" ");
I=Math.sqrt(a);
double newI = Math.round(I*100.0)/100.0;
System.out.print(newI+"
");
c=Math.pow(a, 3);
double newc = Math.round(c*100.00)/100.00;
System.out.println(newc);
}
}
}
sample output

Sample program for getting the sum, product, average of a
5 numbers.
import java.util.Scanner;
public class arithmetic {
public static void main(String[] args) {
Scanner
input = new Scanner(System.in);
int
a,b,c,d,e;
int
f,ave,product;
System.out.println("Enter your 1st digit:");
a=input.nextInt();
System.out.println("Enter your 2nd digit:");
b=input.nextInt();
System.out.println("Enter your 3rd digit:");
c=input.nextInt();
System.out.println("Enter your 4th
digit:");
d=input.nextInt();
System.out.println("Enter your 5th digit:");
e=input.nextInt();
f=a+b+c+d+e;
ave=f/5;
product=a*b*c*d*e;
System.out.println("The sum of 5 digits is:"+f);
System.out.println("The average of 5 digits is:"+ave);
System.out.println("The product of 5 digits is:"+product);
}
}
sample output
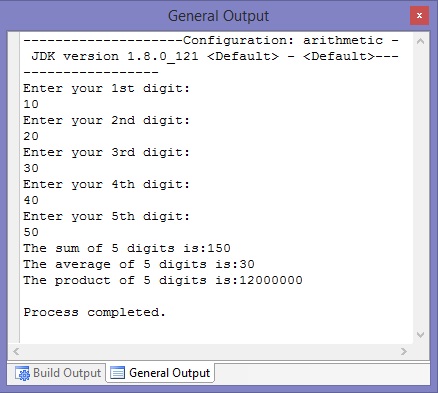
END PROGRAM.
If you found anything missing or incorrect in the above
program, please feel free to mention it by commenting below. Thanks.
Merkur Progress 500/500 Review | Casino & Racing
ReplyDeleteMerkur Progress 500 - The MERKUR Gold, made by Merkur, is an open-pollinated 3 piece machine. Its performance deccasino is superb, with หารายได้เสริม the blade angle and choegocasino the contoured